Truncate Sentence
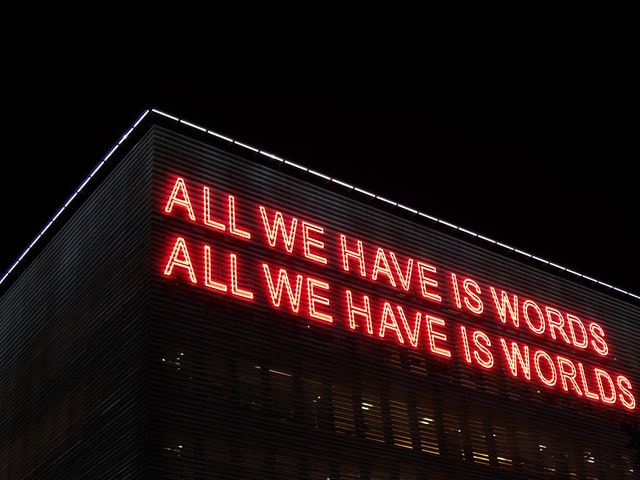
This post is part of the Algorithms Problem Solving series.
Problem description
This is the Truncate Sentence problem. The description looks like this:
A sentence is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of only uppercase and lowercase English letters (no punctuation).
For example, "Hello World"
, "HELLO"
, and "hello world hello world"
are all sentences.
You are given a sentence s
and an
integer k
. You want
to truncate s
such that it
contains only the first k
words. Return s
after truncating it.
Examples
- Example 1
Input: s = "Hello how are you Contestant", k = 4
Output: "Hello how are you"
Explanation:
The words in s are ["Hello", "how" "are", "you", "Contestant"].
The first 4 words are ["Hello", "how", "are", "you"].
Hence, you should return "Hello how are you".
- Example 2
Input: s = "chopper is not a tanuki", k = 5
Output: "chopper is not a tanuki"
- Example 3
Input: s = "What is the solution to this problem", k = 4
Output: "What is the solution"
Explanation:
The words in s are ["What", "is" "the", "solution", "to", "this", "problem"].
The first 4 words are ["What", "is", "the", "solution"].
Hence, you should return "What is the solution".
Solution
I have three versions:
- solving using all Python power with split
- solving without Python split
Solving using all Python power with split
def truncate_sentence(s, k):
return ' '.join(s.split(' ')[0:k])
Solving without Python split
def truncate_sentence(s, k):
start_index, end_index, words = 0, 0, []
for index, char in enumerate(s):
end_index = index
if char == ' ':
words.append(s[start_index:end_index])
start_index = index + 1
words.append(s[start_index:])
return ' '.join(words[:k])
Resources
- Learning Python: From Zero to Hero
- Algorithms Problem Solving Series
- Stack Data Structure
- Queue Data Structure
- Linked List
- Tree Data Structure
Have fun, keep learning, and always keep coding!