Deepest Leaves Sum
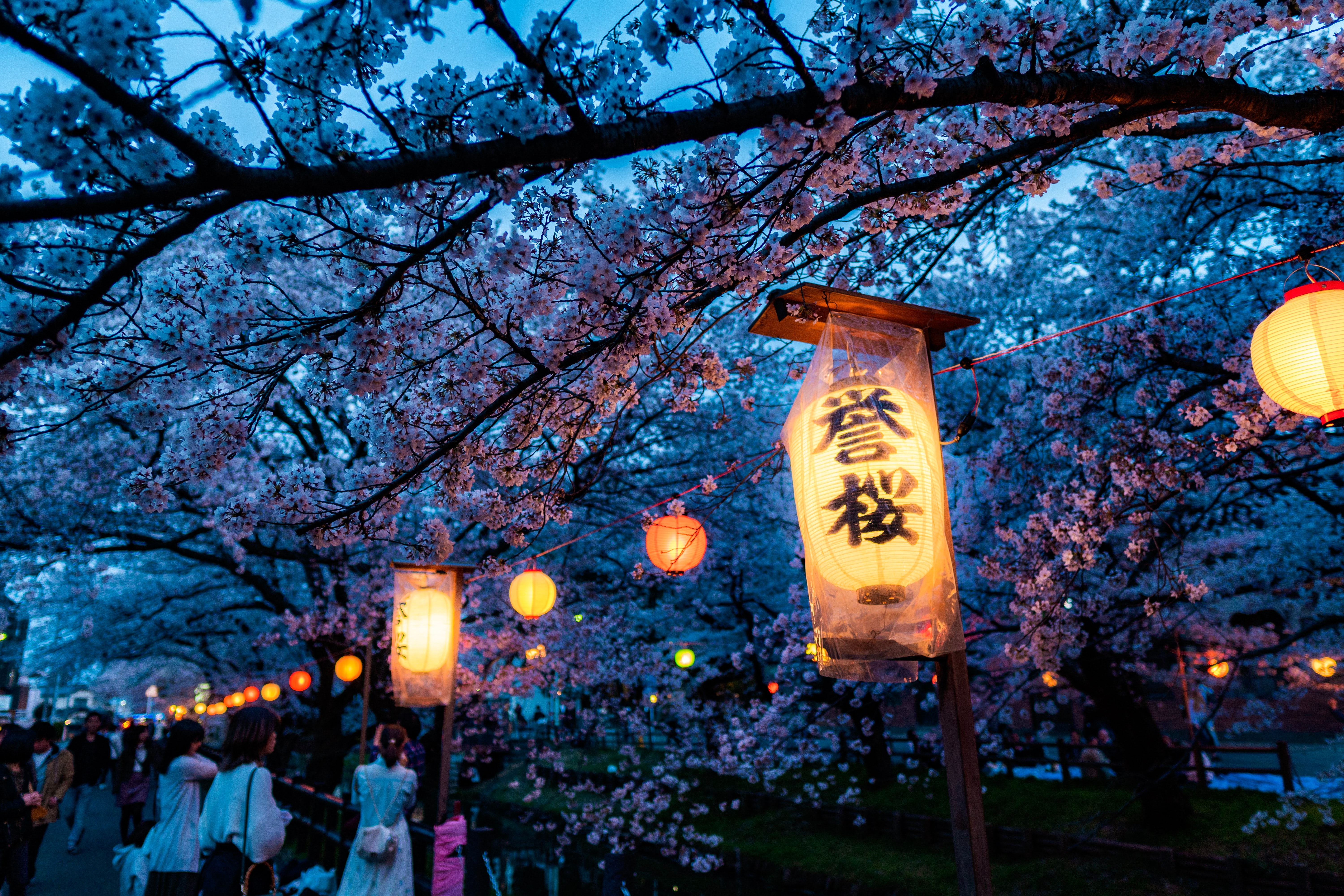
This post is part of the Algorithms Problem Solving series.
Problem description
This is the Deepest Leaves Sum problem. The description looks like this:
Given a binary tree, return the sum of values of its deepest leaves.
Examples
Input: root = [1,2,3,4,5,null,6,7,null,null,null,null,8]
Output: 15
Solution
My first idea was to organize the tree node values into a hash map. The tree's level as the key and a list of node values as the value.
For this tree example
10 ---> level 1
/ \
5 20 ---> level 2
/ \ / \
2 7 15 22 ---> level 3
The hash map will look like this:
{
1: [10],
2: [5, 20],
3: [2, 7, 15, 22]
}
- The first level is only the value 10
- The second level is a lista of 5 and 20
- And the third level is 2, 7, 15, and 22
We build this with a helper function.
def helper(node, mapper, level):
if level in mapper:
mapper[level] = mapper[level] + [node.val]
else:
mapper[level] = [node.val]
if node.left:
mapper = helper(node.left, mapper, level + 1)
if node.right:
mapper = helper(node.right, mapper, level + 1)
return mapper
The first thing is to verify if the level is in the mapper. If it is, add the current node's value into the list for this level. Otherwise, just set a list with only the current value.
Then traverse the list to the left if it has a left child. And traverse to the right if it has a right child.
We finish the helper algorithm by returning the mapper. Now we have the map with all the tree data separate by level.
We just need to get the deepest level from this map, get the list from the map, and then sum all the values from the list.
def sum_deepest_leaves(root):
mapper = helper(root, {}, 1)
deepest_level = 1
for level in mapper:
if level > deepest_level:
deepest_level = level
deepest_level_nodes_values = mapper[deepest_level]
nodes_values_sum = 0
for node_value in deepest_level_nodes_values:
nodes_values_sum += node_value
return nodes_values_sum
Resources
- Learning Python: From Zero to Hero
- Algorithms Problem Solving Series
- Stack Data Structure
- Queue Data Structure
- Linked List
- Tree Data Structure
Have fun, keep learning, and always keep coding!