Shuffle the array
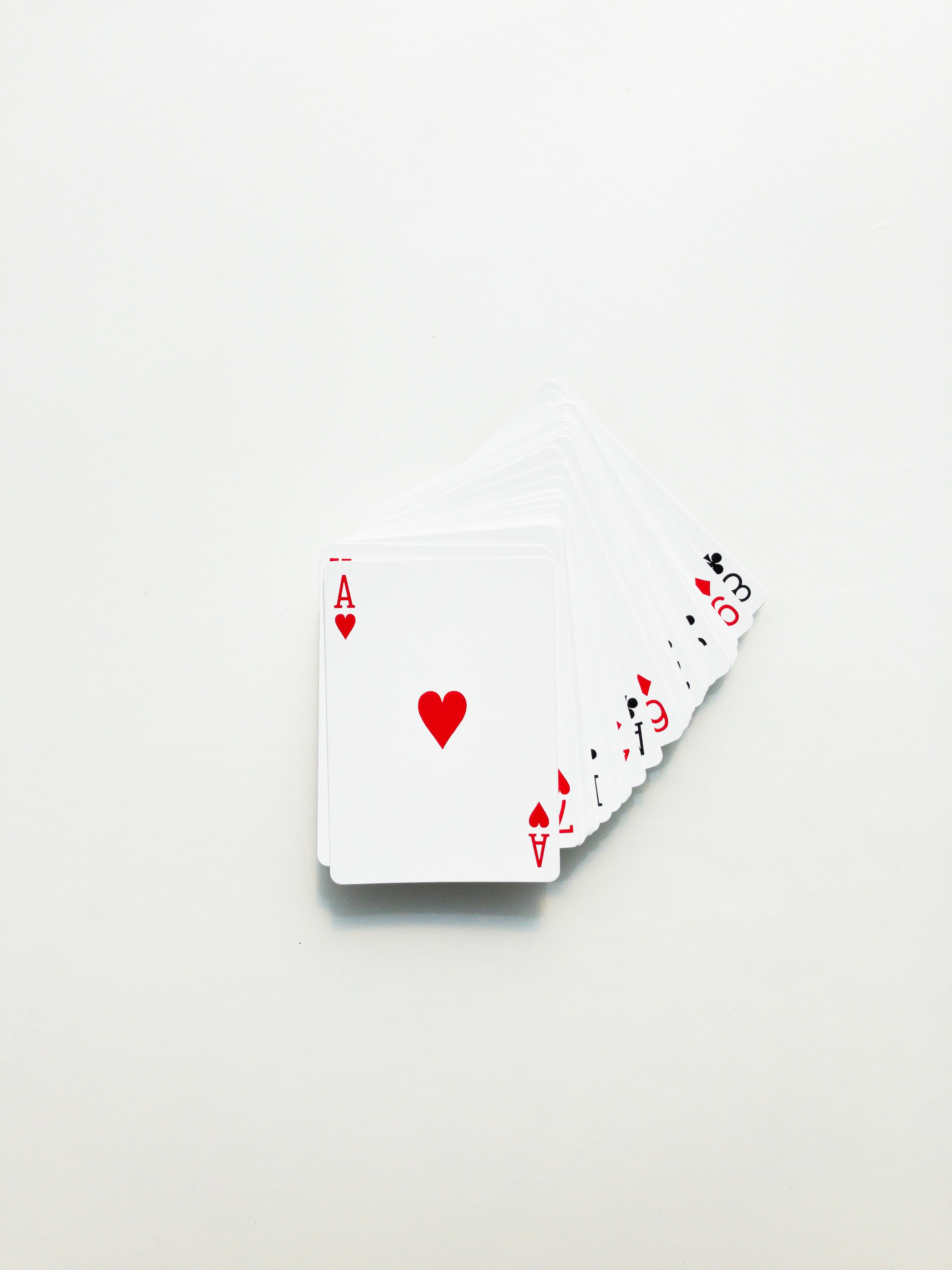
This post is part of the Algorithms Problem Solving series.
Problem description
This is the Shuffle the array problem. The description looks like this:
Given the array nums
consisting
of 2n
elements in the
form [x1,x2,...,xn,y1,y2,...,yn]
.
Return the array in the form [x1,y1,x2,y2,...,xn,yn]
.
Examples
Input: nums = [2,5,1,3,4,7], n = 3
Output: [2,3,5,4,1,7]
Input: nums = [1,2,3,4,4,3,2,1], n = 4
Output: [1,4,2,3,3,2,4,1]
Input: nums = [1,1,2,2], n = 2
Output: [1,2,1,2]
Solution
The idea of my solution was to get the first and the last half of the
nums
list. Then iterate through the lists and append one
by one.
def shuffle(nums, n):
first_half = nums[:n]
last_half = nums[n:]
final_list = []
for index in range(len(first_half)):
final_list.append(first_half[index])
final_list.append(last_half[index])
return final_list
We could also iterate through the lists simultaneously by using the
zip
function. And append the items in one call, instead
of two lines:
def shuffle(nums, n):
first_half = nums[:n]
last_half = nums[n:]
final_list = []
for first, last in zip(first_half, last_half):
final_list.extend((first, last))
return final_list
Resources
- Learning Python: From Zero to Hero
- Algorithms Problem Solving Series
- Stack Data Structure
- Queue Data Structure
- Linked List
- Tree Data Structure
Have fun, keep learning, and always keep coding!