to Lower case
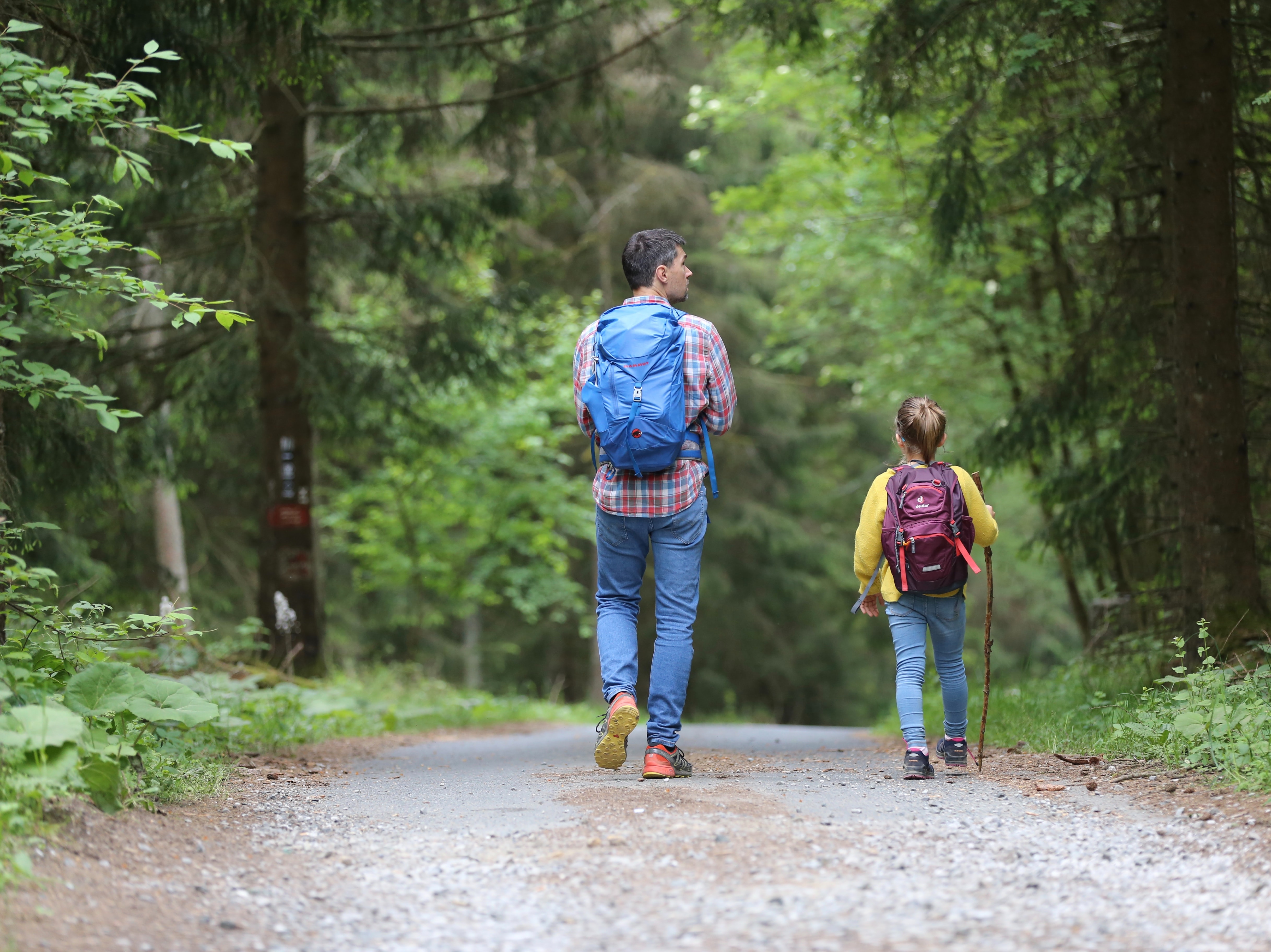
This post is part of the Algorithms Problem Solving series.
Problem description
This is the To Lower Case problem. The description looks like this:
Implement function ToLowerCase() that has a string parameter str, and returns the same string in lowercase.
Examples
Example 1:
Input: "Hello"
Output: "hello"
Example 2:
Input: "here"
Output: "here"
Example 3:
Input: "LOVELY"
Output: "lovely"
Solution
My solution to this problem was to iterate through the entire string,
and for each character, I append its lower case version. To verify if
it needs to transform the character to lower case, I simply get the
integer representation of the Unicode character by using the
ord
function. If it is between 65 and 90, both inclusive,
the character is capitalized, so I need to transform it to lower case.
To transform to lower case, I just need to:
- Transform to the Unicode integer
- Sum 32
- Transform back to the character representation of the Unicode
After the process of each character, I have my list of lower case characters. Then, I just need to join all the characters to get my final string back.
def lower_case_char(char):
if ord(char) >= 65 and ord(char) <= 90:
return chr(ord(char) + 32)
return char
def lower_case(str):
list_of_chars = []
for char in str:
list_of_chars.append(lower_case_char(char))
return ''.join(list_of_chars)
Resources
- Learning Python: From Zero to Hero
- Algorithms Problem Solving Series
- Stack Data Structure
- Queue Data Structure
- Linked List
- Tree Data Structure
Have fun, keep learning, and always keep coding!